Using Injected Providers in a React App with ZKsync and Web3js
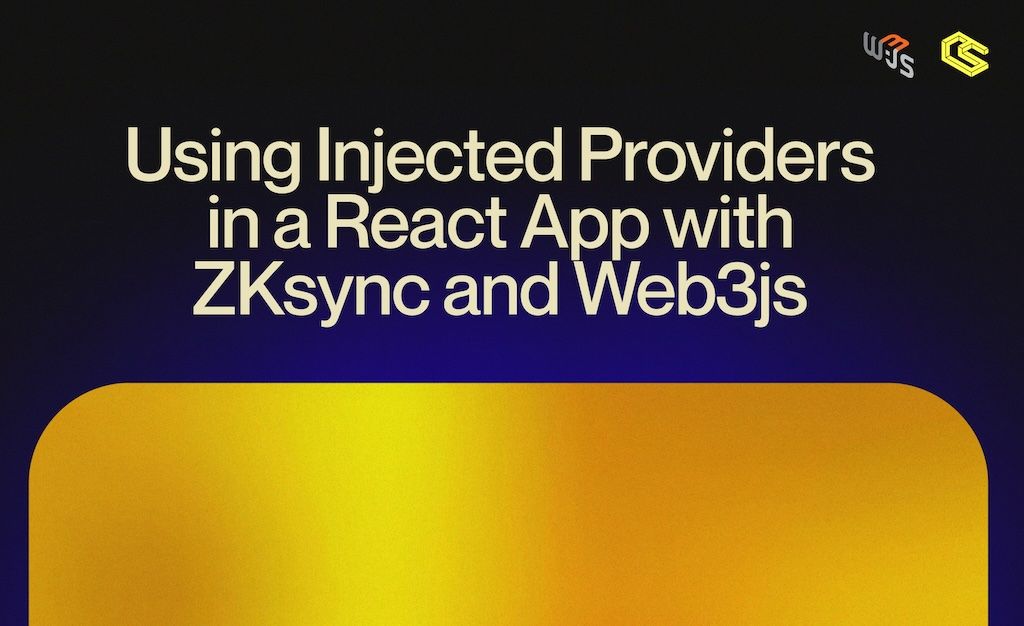
In this article, we will explore how to set up a Next.js application to interact with the ZKsync network using an injected provider, such as MetaMask. We’ll build a basic application that connects to a user’s wallet, retrieves account information and sends a small amount of ETH to a randomly generated address.
Setting Up Your Project
We’ll start by setting up a simple Next.js project. If you haven’t already set up a Next.js environment, you can do so by running:
Next, install the necessary dependencies:
The web3
the library will allow us to interact with Ethereum-based networks and the web3-plugin-zksync
enables us to work with ZKsync.
Example Code: Connecting with an Injected Provider
Here’s the full example code for connecting with an injected provider in a Next.js application:
Code Breakdown
Initializing Web3 and ZKSync Plugin
In the useEffect
hook, we create an instance of Web3
and register the ZKsync plugin using the injected provider (window.ethereum
). This allows us to interact with the user's wallet and blockchain from the browser.
Connecting to the Wallet
When the “Connect to the wallet” button is clicked, we use web3.ZKsync.L2.eth.requestAccounts()
to prompt the user to connect their wallet. Once connected, we store the main account's address in the state.
Retrieving the Balance
The handleGetBalance
function fetches the balance of the connected account using web3.ZKsync.L2.getBalance()
.
Sending ETH
In the handleSendSomewhere
function, we generate a random account, construct a transaction, and send it using web3.ZKsync.L2.eth.sendTransaction()
. We then display the transaction receipt, including a link to the block explorer to check the transaction.
Conclusion
In this article, we’ve demonstrated how to set up a basic Next.js application that connects to a user’s wallet using an injected provider like MetaMask. We covered connecting to the wallet, retrieving balances, and sending transactions using ZKsync.
Helpful Links and Resources
Here are the essential links to help you get started:
- Web3.js Plugin for ZKsync: You can find the official plugin repository here.
- Examples for Using the Plugin: I've also prepared a dedicated repository with all the code examples from this tutorial and more, which you can access here.
- ZKsync Web3.js Documentation: For more detailed information and resources, check out the official documentation here.