Test Your TypeScript Code With Karma and Jest
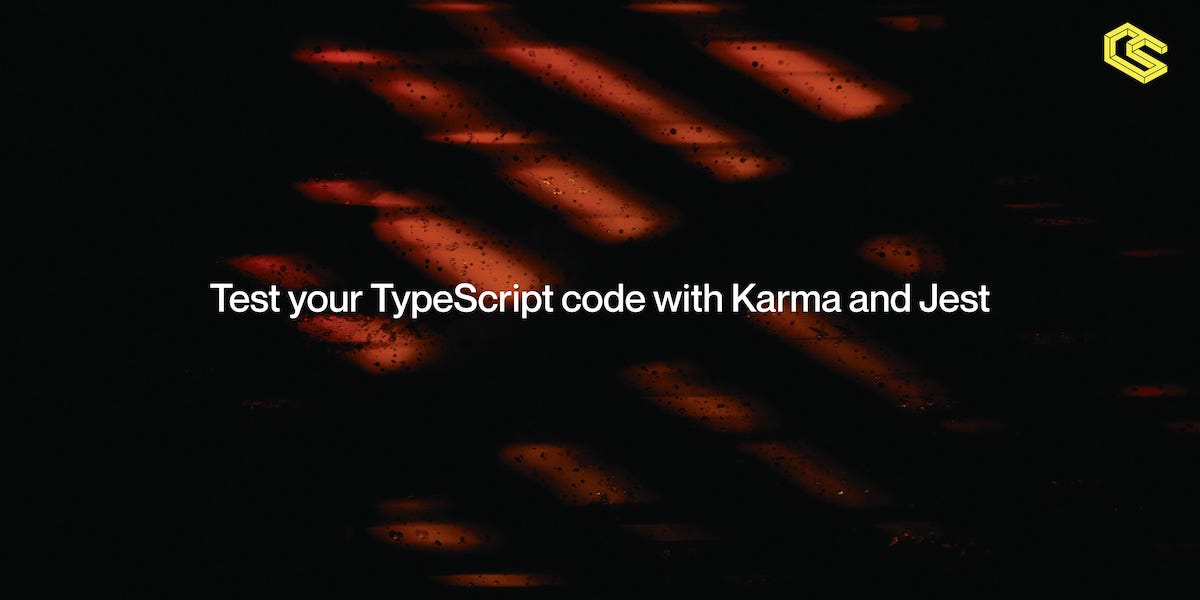
Build comprehensive test coverage for your TS code with this simple coding tutorial
This article is about how you can cover your code with tests and run them on the server and in the browser. We will create a library and cover it with tests. Before we begin, let's go over some basics.
Software testing is a quintessential practice in software development. Oftentimes, tests that consume a lot of time (such as regression testing) are done through automated tests. This way, the efforts of a software engineer can be directed at higher-value tasks. And within the automated testing bucket, there are unit tests (scrutinizing the smallest testable parts of an application), integration tests (ensuring individual modules are combined and tested in a group), and system tests (testing the system as a whole). In TypeScript (TS), we have numerous testing frameworks to design and document our test cases to ensure our code is properly covered. One of the most popular frameworks is Jest. which by default is only for the server. However, oftentimes this is not enough as in the case when you need to build test coverage in different browser environments. In such instances, developers might need to have the know-how to leverage different frameworks in an integrated fashion to compile comprehensive coverage for their code.
Let's start 👇
Let's imagine that we're developing a library in TypeScript that should work in both the browser and the server.
Of course, we will cover our code with tests. In our example, we will use Jest, one of the most popular testing frameworks. However, to avoid writing tests separately for both the browser and the server, we can use a framework that can run the same tests for both instances simultaneously. One option is Karma.
First, we will write a pseudo library, which we will cover with tests. I think now it's more relevant to write in TypeScript, so we'll write the library in it.
Our library connects via WebSockets to the server. Therefore, it's necessary to make a test WebSocket server.
Full code on Github
Let's write some tests using the Jest framework and TypeScript. Let's install it first.
yarn add @types/jest jest jest-extended
And then add some integration tests.
Let's try running our code.
jest - config=./test/jest.config.js
Fine! The tests are working. But these tests are run on the server. Let's make the same tests run from a browser, and even better, from several browsers. To do this, install Karma:
yarn add Karma -D
Karma has a lot of extensions. In our case, we will need to install some of them.
Since Karma does not support Jest by default, we will use a more Jest-like testing library - Jasmine. So install the Jasmine framework itself.
yarn add jasmine -D
We need to add virtual browser modules to run the tests in browsers.
yarn add karma-chrome-launcher karma-firefox-launcher -D
Modules for compiling code.
yarn add karma-webpack ts-jest ts-loader webpack -D
Add the karma.config.js file. In this file, we can configure Karma, specify which files to run, how to compile the code, and much more. More details about each setting item are here.
So in the settings, we indicated:
-
which files we have as tests;
-
what settings for compiling TypeScript;
-
and which folder.
To teach Karma to support Jest methods, we will use this trick. Let's create a karma.setup.js file with the following content:
Additionally, I've added support for a relatively new javascript feature: setImmediate.
global.setImmediate = global.setImmediate || ((fn, ...args) => global.setTimeout(fn, 0, ...args));
window.setImmediate = global.setImmediate;
Add a call of this file to preprocessors before calling the main script and to files to initialize additional methods and functions.
Trying to run.
karma start karma.config.js
Conclusion
Because Karma does not support Jest out-of-the-box, we must build a workaround. Today, we covered one of these workarounds by showing you how to set up Karma with Jest and TypeScript.
What tools do you use for testing in the browser and on the server? Let us know in the comments 👇
The complete code of this experiment you can find here.
About ChainSafe
ChainSafe is a leading blockchain research and development firm specializing in infrastructure solutions for the decentralized web. Alongside client implementations for Ethereum, Polkadot, Filecoin, and Mina, we're building a portfolio of web3 products - Files, Storage, web3.unity, and more. As part of our mission to build innovative products for users and better tooling for developers, ChainSafe embodies an open source and community-oriented ethos. To learn more, click here.