Mastering Web3.js utilities
The web3.js utilities package is one of the most widely used packages of the web3.js library (~920k downloads a month). If you’re developing a dApp or honing your skills, this article will demonstrate how to use these functions to their full potential.
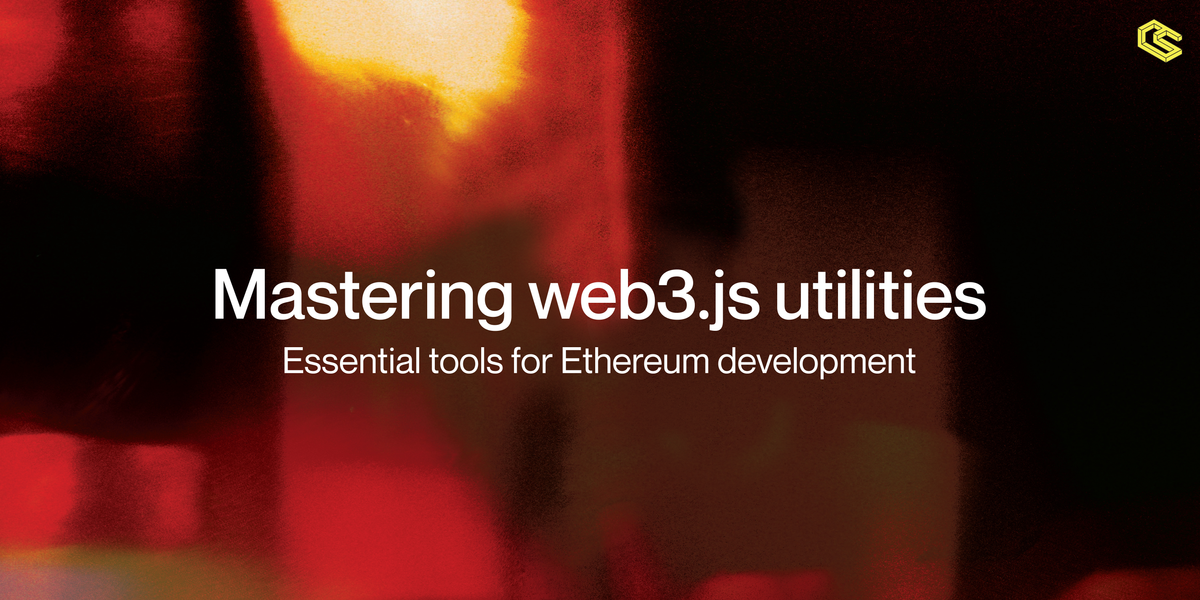
The web3.js utilities pack is one of the most widely used packages of the web3.js library. If you’re developing a dApp or honing your skills, this article will demonstrate how to use these functions to their full potential.
In 2020, ChainSafe was selected by the Ethereum Foundation to maintain and further develop the web3.js library. You can find more information in our original announcement. If you have any questions about web3.js, we’re happy to help out on both Discord and Twitter.
Code examples used in this article can be found in our GitHub repo. You can also follow along with our video tutorial 🙂
Imports
Enter the code below from getting_started.js:
const { Web3 } = require("web3"); //OR -> import { Web3 } from “web3”
const web3 = new Web3("https://eth.llamarpc.com");
async function main() {
const blockNumber = await web3.eth.getBlockNumber();
console.log(blockNumber);
}
main();
There are three ways to import the .utils package. We’ll use wei as an example.
Import using a provider
- Import the web3.js module (web3.js library)
- Initialize the provider (link to a note)
- Access the package by typing web3.utils.toWei
Import .utils from the web3
- Import .utils from the web3 module
- Use any function using .utils.toWei
Import a specific function
- Enter: const { Web3 } = require("web3-utils");
- The specific function is now usable
RandomBytes()
There are two possible formats for generating RandomBytes: Array and Hexadecimal.
If you don’t send any arguments, the byte value for each command is set to 32.
Array format
const randomBytes32 = utils.randomBytes(32);
console.log(randomBytes32);
Hexadecimal format
const randomHex32 = web3.utils.randomHex(32);
console.log(randomHex32);
Conversions
Ethereum denominations
There are two functions to perform conversions with Ethereum denominations: toWei and fromWei.
Function: toWei
The function toWei can receive any type of unit and will always return wei.
To convert 1 WEI to ETH:
const oneEther = web3.utils.toWei("1", "ether");
// 1_000_000_000_000_000_000
Function: fromWei
The function fromWei will always receive wei and can return any type of value.
const oneWei = web3.utils.fromWei("1", "ether");
//0.000000000000000001
Hex values
There are several ways to convert any value into a hexadecimal value, but the easiest way is to use the function toHex.
toHex can convert hexadecimal values from:
Numbers
console.log(utils.tohex(10));
BigInt
console.log(utils.tohex(10n));
Strings
console.log(utils.tohex(“10”));
Booleans
console.log(utils.tohex(false));
Objects
console.log(utils.tohex({ name: santiago }));
The alternative methods to convert a number to a hexadecimal value are:
FromNumber
console.log(utils.numberToHex(10));
FromDecimal
console.log(utils.fromDecimal(10));
FromUTF8
console.log(utils.utf8ToHex(10));
FromASCII
console.log(utils.asciiToHex(10));
Converting bytes in array format
There are two functions we can use to convert bytes in array format. First, create an array. For example:
const arr = [72,12]
Using an array as a parameter:
console.log(utils.toHex(arr))
This will read the array as a Uint8 array.
To send this array as bytes, use the function:
console.log(utils.bytesToHex(arr));
UTF & ASCII
We can convert hexadecimal values to UTF and ASCII values. We recommend using UTF for a broader range of characters and emoji support. For example, we’ll run this emoji 🙂 as a hexadecimal value.
UTF
console.log(“utf”, utils.toUtf8(“0xf09f088a”));
ASCII
console.log(“ascii”, utils.toAscii(“0xf09f088a”));
When you run these commands, the UTF returns 🙂 but ASCII returns a somewhat Lovecraftian result.
Numbers & BigInt
There are three functions used to convert hexadecimal values into numbers.
ToNumber
console.log(utils.toNumber(“0xa”));
HexToNumberString
console.log(utils.hexToNumberString(“0xa”));
ToBigInt
console.log(utils.toBigInt(“0xa”));
Hashing Functions
There are two main functions for hashing.
Sha3 will always use a string as a parameter and return the hash in a hexadecimal value:
console.log(utils.sha3(“web3”));
SoliditySha3 can receive a string, uint, address, or bytes as a parameter and will return the hash in a hexadecimal value:
String
console.log(utils.soliditySha3({ type: “string”, value: “web3” }));
Uint
console.log(utils.soliditySha3({ type: “uint256”, value: “123” }));
Address
console.log(utils.soliditySha3({ type: “address”, value: “0x…” }));
Bytes
console.log(utils.soliditySha3({ type: “bytes”, value: [72,12] }));
Addresses
The isAddress() function is currently deprecated. If you want to see if an address is valid, use the function:
console.log(utils.ChecksumAddress(“0x…”));
Passing an invalid address returns “Error: invalid ethereum address.”
Passing an address with all characters lowercase will return the corrected address.
To learn more about Checksum address function, see ERC-55 checksum address encoding.
Packing and Padding
Packing
The ecodePacked function behaves the same as Solidity’s abi.econdePacked().
Packing Strings
console.log(utils.encodePacked(“10”, “10”, “10”));
This will pack the string and return a hex value.
Packing Numbers
console.log(utils.encodePacked(10, 10, 10));
This will pack the numbers, treating them as a Uint256, converting them to a 32byte number, then pack the different values.
Padding
There are two main functions we can use for Padding: PadRight and PadLeft.
Parameters: number, number of characters to receive after padding.
PadRight
console.log(utils.padRight(10, 6));
Result: 0xa00000
PadLeft
console.log(utils.padLeft(10, 6));
Result: 0x00000a
The third (and optional) parameter is the character or number you want to use to pad your input.
Example:
console.log(utils.padRight(10, 6, “x”));
Result: 0xaxxxxx
Compare Block Numbers
The final function is compareBlockNumbers:
console.log(utils.compareBlockNumbers(“pending”, “last”));
If the first argument is higher than the second, it will return 1.
If the first argument is lower than the second, it will return -1.
If both arguments are equal, it will return a 0 (zero).
Learn more about web3.js utilities
If you want to explore web3.js utilities further, all functions with code examples can be found in the web3.js docs:
👉 Follow @web3_js on Twitter for updates!
Still have questions? You can connect with other developers and get web3.js support in the ChainSafe Discord channel.
Just go to #web3js-general for all your web3.js questions. We hope to see you there soon! 😀
About ChainSafe
ChainSafe is a leading blockchain research and development firm specializing in protocol engineering, cross-chain interoperability, and web3 gaming. Alongside its contributions to major ecosystems such as Ethereum, Polkadot, and Filecoin, ChainSafe creates solutions for developers across the web3 space utilizing expertise in gaming, interoperability, and decentralized storage. As part of its mission to build innovative products for users and improved tooling for developers, ChainSafe embodies an open-source and community-oriented ethos to advance the future of the internet.
Website | Twitter | Linkedin | GitHub | Discord | YouTube | Newsletter