Integrating web3.js into the Frontend
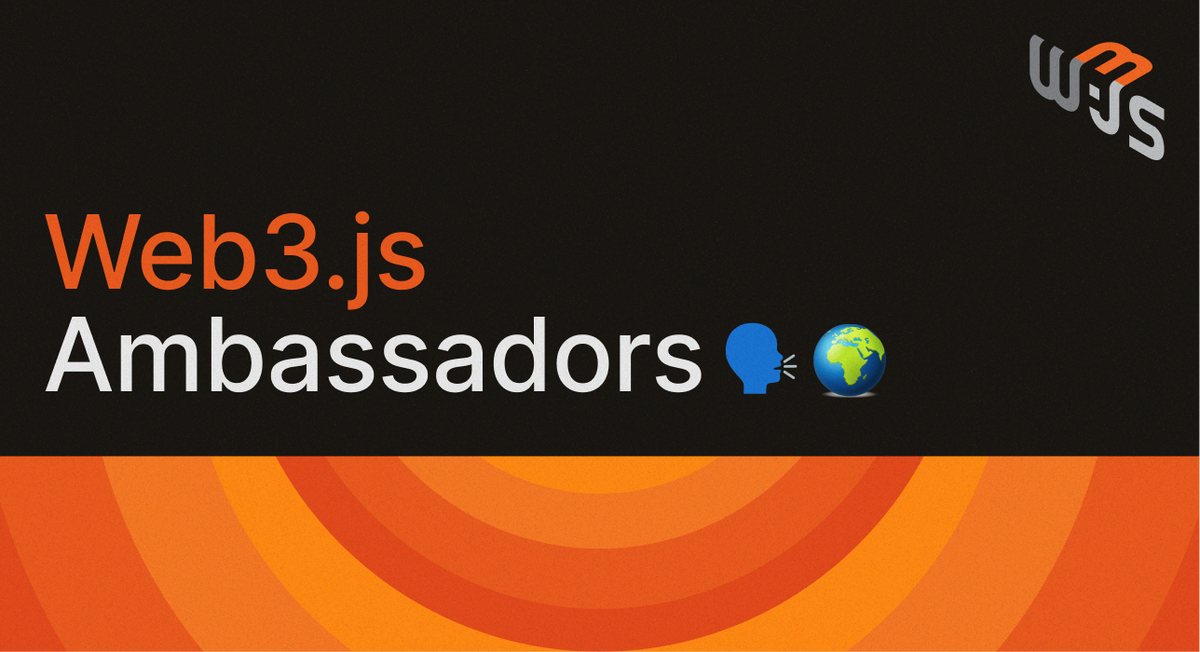
Integrating web3 capabilities into the frontend is crucial for building decentralized applications (dApps) that interact seamlessly with the blockchain. This blog covers the essentials of using web3.js, connecting to the Ethereum network, and building a simple dApp.
Introduction to web3.js
Web3.js is a JavaScript library for interacting with EVM (Ethereum virtual machine) blockchains. It provides functionalities for sending transactions, interacting with smart contracts, and retrieving blockchain data.
Key features
- Connection management: Connect to Ethereum nodes via HTTP or WebSocket.
- Smart contract interaction: Deploy and interact with smart contracts.
- Transaction management: Create, sign, and send transactions.
- Account management: Manage Ethereum accounts and wallets.
Installation
To install web3.js, use npm or yarn:
npm install web3
# or
yarn add web3
Basic usage
const {Web3} = require('web3');
const web3 = new Web3('<http://localhost:8545>'); // Connect to a local Ethereum node
web3.eth.getAccounts().then(console.log); // List all accounts
Connecting to Ethereum
Connecting to the Ethereum network is the first step in interacting with the blockchain. You can connect to different types of networks such as mainnet, testnet, or local development networks.
Connecting to mainnet (e.g., Base)
const web3 = new Web3('https://base.llamarpc.com');
Connecting to testnet (e.g., Sepolia)
const web3 = new Web3('https://ethereum-sepolia-rpc.publicnode.com');
Connecting to local development networks
const web3 = new Web3('<http://localhost:8545>');
These endpoints are free and recommended ONLY for testing and not production.
Interacting with smart contracts
To interact with smart contracts, you need the contract's ABI (Application Binary Interface) and its address on the blockchain.
Deploying a smart contract
const contractABI = [...] // ABI array
const contractBytecode = '0x...' // Bytecode
const deploy = async () => {
const accounts = await web3.eth.getAccounts();
const contract = new web3.eth.Contract(contractABI);
contract.deploy({
data: contractBytecode
})
.send({
from: accounts[0],
gas: 1500000,
gasPrice: '30000000000'
})
.then((newContractInstance) => {
console.log(newContractInstance.options.address); // Instance with the new contract address
});
};
deploy();
Interacting with an existing contract
const contractAddress = '0xYourContractAddress';
const contract = new web3.eth.Contract(contractABI, contractAddress);
// Call a contract method
contract.methods.yourMethod().call().then(console.log);
// Send a transaction to a contract method
contract.methods.yourMethod().send({ from: '0xYourAccountAddress' });
Building a simple dApp
In this example, we'll also create a dApp to connect user accounts to an Ethereum provider injected as MetaMask.
This dApp is a simple demonstration of how to connect to the Ethereum network and interact with user accounts, providing a basis for the development of more complex decentralized applications.
Setting Up the frontend
- Create an HTML file
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple dApp</title>
</head>
<body>
<h1>My Simple dApp</h1>
<button id="getAccounts">Get Accounts</button>
<div id="accounts"></div>
<script src="<https://cdn.jsdelivr.net/npm/web3@1.3.6/dist/web3.min.js>"></script>
<script src="app.js"></script>
</body>
</html>
- Create an app.js file
window.addEventListener('load', async () => {
// Modern dapp browsers...
if (window.ethereum) {
window.web3 = new Web3(window.ethereum);
try {
// Request account access if needed
await window.ethereum.enable();
// Acccounts now exposed
} catch (error) {
console.error('User denied account access');
}
}
// Legacy dapp browsers...
else if (window.web3) {
window.web3 = new Web3(web3.currentProvider);
}
// Non-dapp browsers...
else {
console.log('Non-Ethereum browser detected. You should consider trying MetaMask!');
}
const web3 = window.web3;
document.getElementById('getAccounts').addEventListener('click', () => {
web3.eth.getAccounts().then(accounts => {
document.getElementById('accounts').innerText = accounts.join('\\\\n');
});
});
});
Explanation
- HTML file: Sets up a basic web page with a button to get accounts.
- JavaScript file: Connects to the Ethereum network and retrieves accounts using Web3.js.
After covering the fundamentals of integrating blockchain access into the frontend of a dApp using web3.js, you should be able to connect to the Ethereum network, interact with smart contracts, and build basic applications.
Learn more about web3.js
If you want to explore web3.js further, all functions with code examples can be found in the web3.js docs:
👉 Visit web3.js docs
👉 Follow @web3_js on Twitter for updates!
Still have questions? You can connect with other developers and get web3.js support in the ChainSafe Discord channel.
Just go to #web3js-general for all your web3.js questions. We hope to see you there soon! 😀
👉 Join us on Discord!
About ChainSafe
ChainSafe is a leading blockchain research and development firm specializing in protocol engineering, cross-chain interoperability, and web3 gaming. Alongside its contributions to major ecosystems such as Ethereum, Polkadot, and Filecoin, ChainSafe creates solutions for developers across the web3 space utilizing expertise in gaming, interoperability, and decentralized storage. As part of its mission to build innovative products for users and improved tooling for developers, ChainSafe embodies an open-source and community-oriented ethos to advance the future of the internet.
Website | Twitter | Linkedin | GitHub | Discord | YouTube | Newsletter